Dev Urandom Example C
I'm trying to read an eight byte number from /dev/urandom, but i want the number to be in hexademical. For example: Code: char.bytes; bytes = (char.)m reading from /dev/urandom in c Share your knowledge at the LQ Wiki. Dec 29, 2015 If you run 'make load' or reboot, the kernel module will be loaded into the running kernel, but now will replace the /dev/urandom device file. The old /dev/urandom device is renamed (keeping it's inode number). This allows any running process that had /dev/urandom to continue running without issues. This means that the call will not block, but the output may contain less entropy than the corresponding read from /dev/random. While /dev/urandom is still intended as a pseudorandom number generator suitable for most cryptographic purposes, the authors of the corresponding man page note that, theoretically, there may exist an as-yet-unpublished. I'm looking for ways to use /dev/random (or /dev/urandom) from the command line.In particular, I'd like to know how to use such a stream as stdin to write streams of random numbers to stdout (one number per line). I'm interested in random numbers for all the numeric types that the machine's architecture supports natively.
The difference between the two is that /dev/urandom will always give you some random-looking bits, even if it has to generate extra ones using a cryptographic pseudo-random number generator, while /dev/random will only give you bits that it is confident are in fact random. If your system has a means to generate random numbers involving not only a software algorithm (like the /dev/urandom devices in Unix), then:. Show how to obtain a random 32-bit number from that mechanism.
Hi everyone and good morning :) .
i want to simply read from the linux file 'urandom' a random number and print it out on screen.just simple as that.
this function should be in a file called myrandom.c ( myrandom.h must be made also, which has my function dekleration ) . i will have to compile it later to a static libray and to dynamic library, and then write a main program which prints out 5 random numbers using the static library .
and another main program which prints out 5 random numbers using the dynamic library.
i wrote the myrandom.c code and myrandom.h code i dont know if they are correct .
i tested myrandom.c inside a main program and it gives me random number , but its very big .
ist that the right way to grap a random number from the urandom file?
many thanks
- 5 Contributors
- forum 10 Replies
- 1,212 Views
- 1 Day Discussion Span
- commentLatest Postby zxzLatest Post
Recommended Answers
You're also using printf
incorrectly. When you are printing out the value of an int, printf does not want the address of that int. It wants the int itself.
printf('%d', &rNumber);
is wrong.printf('%d', rNumber);
is right.
WTF is going on with this?
It's still wrong. str
is effectively an int*
, so &str
is an int**
, so *(&str)
is an int*
. So you're doing exactly what you were doing before (wrongly providing an int*
when you …
All 10 Replies
sepp2k378
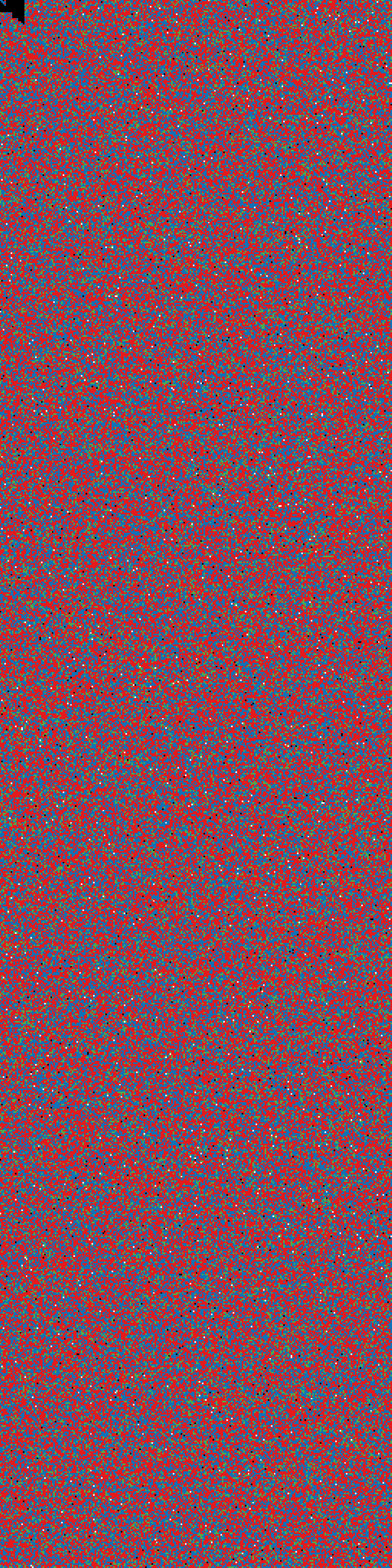
You need to #include <stdio.h>
to get the declaration of fopen
. As it is now you're implicitly declaring fopen
in a way that is incompatible with its definition. You should be getting a warning about that (and in C99 and later you should instead be getting an error that you're using a function without a declaration).
Once you do that, you should be getting another warning that you're implicitly converting the result of fopen
from FILE*
to int
. That's because fopen
doesn't read anything from the file nor does it return an int. fopen
opens the file and then returns a file pointer. That file pointer can then be given to other file IO functions (like fread
, fwrite
, fgets
, fscanf
, fprintf
https://entrancementspaces.weebly.com/blog/windows-81-product-key-generator-2014-free-download. etc.), which will actually read from or write to the file. Once you're done working with the file, you need to close it by passing the file pointer to fclose
.
So no, you're not currently doing it correctly. The number you're printing is simply the numeric value of whichever pointer was returned by fopen
. It has nothing to do with the contents of the file.
Name
random, urandom - kernel random number source devices
Synopsis
#include <linux/random.h>
int ioctl(fd, RNDrequest,param);
Description
The character special files /dev/random and /dev/urandom (present since Linux 1.3.30) provide an interface to the kernel's random numbergenerator. File /dev/random has major device number 1 and minor device number 8. File /dev/urandom has major device number 1 and minor devicenumber 9.
The random number generator gathers environmental noise from device drivers and other sources into an entropy pool. The generator also keeps an estimate ofthe number of bits of noise in the entropy pool. From this entropy pool random numbers are created.
When read, the /dev/random device will only return random bytes within the estimated number of bits of noise in the entropy pool. /dev/randomshould be suitable for uses that need very high quality randomness such as one-time pad or key generation. When the entropy pool is empty, reads from/dev/random will block until additional environmental noise is gathered.
A read from the /dev/urandom device will not block waiting for more entropy. As a result, if there is not sufficient entropy in the entropy pool, thereturned values are theoretically vulnerable to a cryptographic attack on the algorithms used by the driver. Knowledge of how to do this is not available inthe current unclassified literature, but it is theoretically possible that such an attack may exist. If this is a concern in your application, use/dev/random instead.
Writing to /dev/random or /dev/urandom will update the entropy pool with the data written, but this will not result in a higher entropy count.This means that it will impact the contents read from both files, but it will not make reads from /dev/random faster.
Usage
If you are unsure about whether you should use /dev/random or /dev/urandom, then probably you want to use the latter. As a general rule,/dev/urandom should be used for everything except long-lived GPG/SSL/SSH keys.If a seed file is saved across reboots as recommended below (all major Linux distributions have done this since 2000 at least), the output iscryptographically secure against attackers without local root access as soon as it is reloaded in the boot sequence, and perfectly adequate for networkencryption session keys. Since reads from /dev/random may block, users will usually want to open it in nonblocking mode (or perform a read withtimeout), and provide some sort of user notification if the desired entropy is not immediately available.
The kernel random-number generator is designed to produce a small amount of high-quality seed material to seed a cryptographic pseudo-random numbergenerator (CPRNG). It is designed for security, not speed, and is poorly suited to generating large amounts of random data. Users should be very economical inthe amount of seed material that they read from /dev/urandom (and /dev/random); unnecessarily reading large quantities of data from this devicewill have a negative impact on other users of the device.
The amount of seed material required to generate a cryptographic key equals the effective key size of the key. For example, a 3072-bit RSA or Diffie-Hellmanprivate key has an effective key size of 128 bits (it requires about 2^128 operations to break) so a key generator only needs 128 bits (16 bytes) of seedmaterial from /dev/random.
While some safety margin above that minimum is reasonable, as a guard against flaws in the CPRNG algorithm, no cryptographic primitive available today canhope to promise more than 256 bits of security, so if any program reads more than 256 bits (32 bytes) from the kernel random pool per invocation, or perreasonable reseed interval (not less than one minute), that should be taken as a sign that its cryptography is not skillfully implemented.
Configuration
/dev/urandom C++
device.The read-only file entropy_avail gives the available entropy. Normally, this will be 4096 (bits), a full entropy pool.
The file poolsize gives the size of the entropy pool. The semantics of this file vary across kernel versions:
Linux 2.4:This file gives the size of the entropy pool in bytes. Normally, this file will have the value 512, but it is writable, and can be changed to anyvalue for which an algorithm is available. The choices are 32, 64, 128, 256, 512, 1024, or 2048.
Linux 2.6:
This file is read-only, and gives the size of the entropy pool in bits. It contains the value 4096.
The file read_wakeup_threshold contains the number of bits of entropy required for waking up processes that sleep waiting for entropy from/dev/random. The default is 64. The file write_wakeup_threshold contains the number of bits of entropy below which we wake up processes that do aselect(2) or poll(2) for write access to /dev/random. These values can be changed by writing to the files.The read-only files uuid and boot_id contain random strings like 6fd5a44b-35f4-4ad4-a9b9-6b9be13e1fe9. The former is generated afresh for eachread, the latter was generated once.
ioctl(2) interface
Here entropy_count is the value added to (or subtracted from) from the entropy count, and buf is the buffer of size buf_size which getsadded to the entropy pool.
Files
/dev/random
/dev/urandom
See Also
mknod(1)
RFC 1750, 'Randomness Recommendations for Security'